Struts 2 ActionError & ActionMessage Example实例介绍,以下是英文的你们可以看明白吗?
Struts 2 ActionError & ActionMessage Example
By mkyong | | Viewed : 169,295 times
A tutorial to show the use of the Struts 2’s ActionError and ActionMessage class.
1. ActionError – is used to send error feedback message to user – display via <s:actionerror/>.
<s:if test="hasActionErrors()">
<div class="errors">
<s:actionerror/>
</div>
</s:if>
2. ActionMessage – is used to send information feedback message to user, display via <s:actionmessage/>.
<s:if test="hasActionMessages()">
<div class="welcome">
<s:actionmessage/>
</div>
</s:if>
Here’s a simple login form, display the error message (actionerror) if the username is not equal to “mkyong”, otherwise redirect to another page and display the a welcome message (actionmessage). In addition, all the label and error messages are retrieve from the resource bundle (properties file).
1. Folder Structure
See this project structure
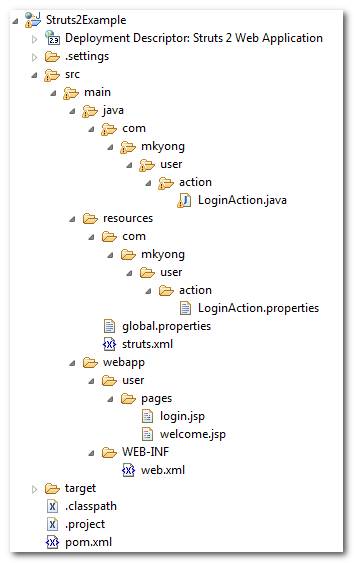
2. Properties file
Two properties files to store the messages.
LoginAction.properties
#Welcome messages
welcome.hello = Hello
#error message
username.required = Username is required
password.required = Password is required
global.properties
#Global messages
global.username = Username
global.password = Password
global.submit = Submit
global.reset = Reset
3. Action
A classic action class, do a simple checking to make sure the username is equal to “mkyong”, and set the error message withaddActionError() or successful message with addActionMessage().
package com.mkyong.user.action;
import com.opensymphony.xwork2.ActionSupport;
public class LoginAction extends ActionSupport{
private String username;
private String password;
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
//business logic
public String execute() {
return "SUCCESS";
}
//simple validation
public void validate(){
if("mkyong".equals(getUsername())){
addActionMessage("You are valid user!");
}else{
addActionError("I don't know you, dont try to hack me!");
}
}
}
4. JSP View
Two simple JSP pages with css style to customize the error message.
login.jsp
<%@ page contentType="text/html; charset=UTF-8" %>
<%@ taglib prefix="s" uri="/struts-tags" %>
<html>
<head>
<style type="text/css">
.errors {
background-color:#FFCCCC;
border:1px solid #CC0000;
width:400px;
margin-bottom:8px;
}
.errors li{
list-style: none;
}
</style>
</head>
<body>
<h1>Struts 2 ActionError & ActionMessage Example</h1>
<s:if test="hasActionErrors()">
<div class="errors">
<s:actionerror/>
</div>
</s:if>
<s:form action="validateUser">
<s:textfield key="global.username" name="username"/>
<s:password key="global.password" name="password"/>
<s:submit key="global.submit" name="submit"/>
</s:form>
</body>
</html>
welcome.jsp
<%@ page contentType="text/html; charset=UTF-8" %>
<%@ taglib prefix="s" uri="/struts-tags" %>
<html>
<head>
<style type="text/css">
.welcome {
background-color:#DDFFDD;
border:1px solid #009900;
width:200px;
}
.welcome li{
list-style: none;
}
</style>
</head>
<body>
<h1>Struts 2 Struts 2 ActionError & ActionMessage Example</h1>
<s:if test="hasActionMessages()">
<div class="welcome">
<s:actionmessage/>
</div>
</s:if>
<h2>
<s:property value="getText('welcome.hello')" /> :
<s:property value="username"/>
</h2>
</body>
</html>
5. struts.xml
Link all together.
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
"http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<constant name="struts.custom.i18n.resources" value="global" />
<package name="user" namespace="/user" extends="struts-default">
<action name="login">
<result>pages/login.jsp</result>
</action>
<action name="validateUser" class="com.mkyong.user.action.LoginAction">
<result name="SUCCESS">pages/welcome.jsp</result>
<result name="input">pages/login.jsp</result>
</action>
</package>
</struts>
6. Run it
http://localhost:8080/Struts2Example/user/login.action
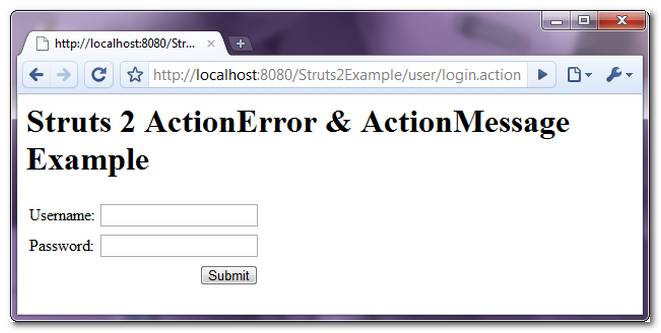
Username is invalid, display error message with <s:actionerror/>
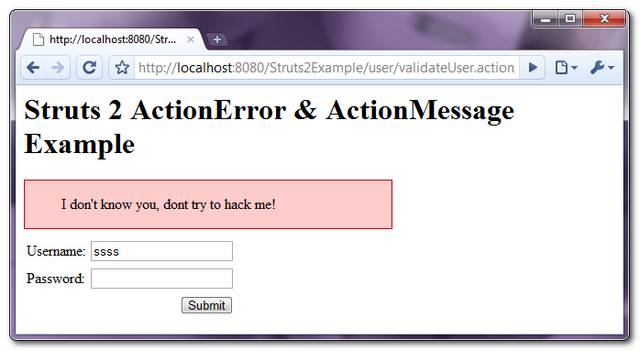
Username is valid, display welcome message <s:actionmessage/>
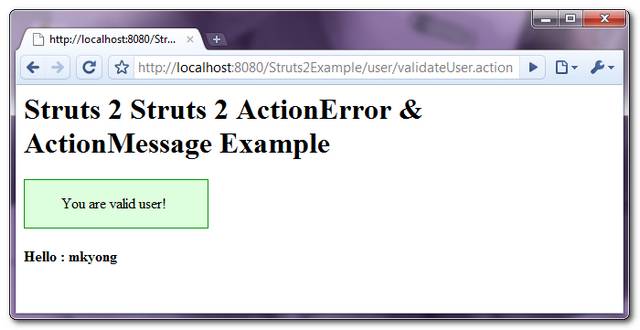